Hey everyone! I made this tutorial and code below to help everyone get quickly started with Azure PlayFab and see how simple it is to use one of their services, the Leaderboards.
1.) Create a PlayFab account: https://developer.playfab.com/en-US/sign-up
2.) Enable API Features:
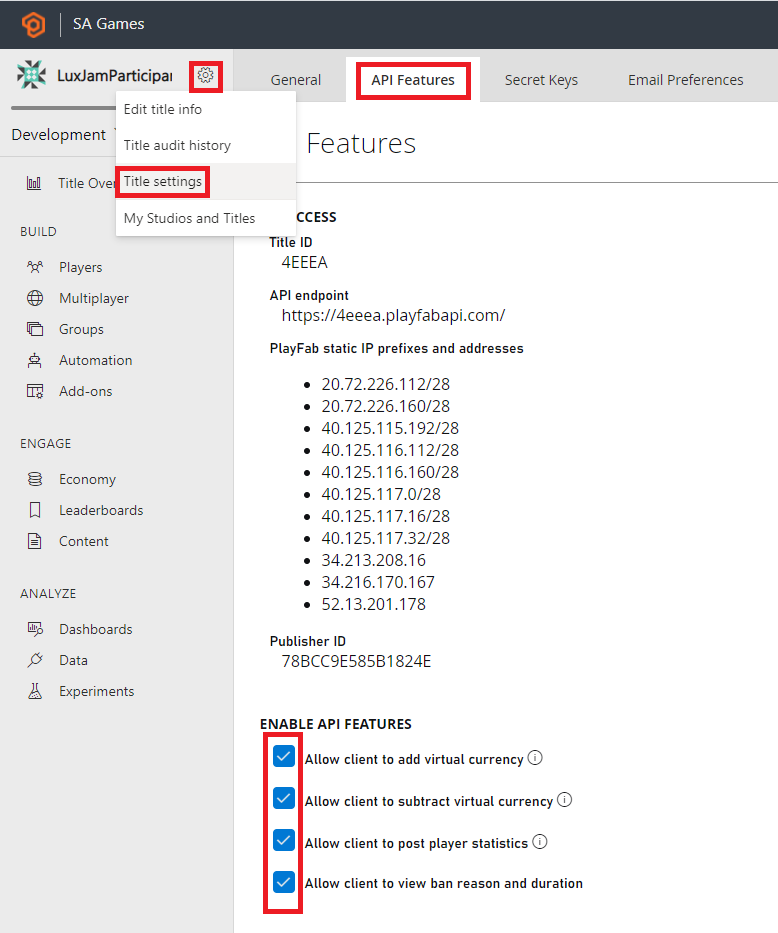
3.) Create a leaderboard
4.) C# class template (custom PlayFab API wrapper):
using System;
using System.Net.Http;
using System.Threading.Tasks;
using System.Text.Json;
using System.Text;
using System.Collections.Generic;
class PlayFab
{
private static readonly HttpClient client = new HttpClient();
private static readonly string titleId = "1EEEA"; // get this from your PlayFab account
private static readonly string leaderboardName = "HighScores"; // get this from your PlayFab account
class AccountResponse {
public class AccountResponseData
{
public string SessionTicket { get; set; }
}
public int code { get; set; }
public AccountResponseData data { get; set; }
}
class LeaderboardResponse
{
public class LeaderboardData
{
public class LeaderboardDataEntry
{
public class LeaderboardDataEntryProfile
{
public string PlayerId { get; set; }
}
public int StatValue { get; set; }
public LeaderboardDataEntryProfile Profile { get; set; }
}
public List<LeaderboardDataEntry> Leaderboard { get; set; }
}
public int code { get; set; }
public LeaderboardData data { get; set; }
}
// create a player's account in your game (cannot be called more than once for same email)
public async Task PlayFabCreateAccountAsync(string email, string password)
{
var raw_response = await client.PostAsync($"https://{titleId}.playfabapi.com/Client/RegisterPlayFabUser", new StringContent($"{{ 'email': '{email}', 'password': '{password}', 'RequireBothUsernameAndEmail': false, 'TitleId': '{titleId}'}}".ToString(), Encoding.UTF8, "application/json"));
AccountResponse response = JsonSerializer.Deserialize<AccountResponse>(await raw_response.Content.ReadAsStringAsync());
if (response.code == 200) Console.WriteLine($"Successful account creation!"); else Console.WriteLine("Account creation failed.");
}
// login
public async Task PlayFabLoginAsync(string email, string password)
{
var raw_response = await client.PostAsync($"https://{titleId}.playfabapi.com/Client/LoginWithEmailAddress", new StringContent($"{{ 'email': '{email}', 'password': '{password}', 'TitleId': '{titleId}'}}".ToString(), Encoding.UTF8, "application/json"));
AccountResponse response = JsonSerializer.Deserialize<AccountResponse>(await raw_response.Content.ReadAsStringAsync());
if (response.code == 200) Console.WriteLine($"Successful login! Your session ID is {response.data.SessionTicket}"); else System.Environment.Exit(1);
client.DefaultRequestHeaders.Add("X-Authorization", response.data.SessionTicket);
}
// add score to leaderboard
public async Task PlayFabAddToLeaderboardAsync(int game_score)
{
var raw_response = await client.PostAsync($"https://{titleId}.playfabapi.com/Client/UpdatePlayerStatistics", new StringContent($"{{ 'Statistics': [ {{ 'StatisticName': '{leaderboardName}', 'Value': {game_score} }} ] }}".ToString(), Encoding.UTF8, "application/json"));
AccountResponse response = JsonSerializer.Deserialize<AccountResponse>(await raw_response.Content.ReadAsStringAsync());
if (response.code == 200) Console.WriteLine($"Successfully added your score {game_score} to the highscores leaderboard!"); else System.Environment.Exit(1);
}
// get leaderboard scores
public async Task PlayFabGetLeaderboardAsync()
{
var raw_response = await client.PostAsync($"https://{titleId}.playfabapi.com/Client/GetLeaderboard", new StringContent($"{{ 'StatisticName': '{leaderboardName}' }}".ToString(), Encoding.UTF8, "application/json"));
var res = await raw_response.Content.ReadAsStringAsync();
LeaderboardResponse response = JsonSerializer.Deserialize<LeaderboardResponse>(res);
if (response.code == 200) Console.WriteLine($"Successfully retrieved {response.data.Leaderboard.Count} leaderboard entries:"); else System.Environment.Exit(1);
response.data.Leaderboard.ForEach(x => Console.WriteLine("\t Player " + x.Profile.PlayerId + " with " + x.StatValue + " points."));
}
}
5.) How to use the template:
PlayFab pf = new PlayFab();
string email = "LanaLuxRox@live.ca";
string password = "d0ntnot1cem3";
int gameScore = 1200;
await pf.PlayFabCreateAccountAsync(email, password);
await pf.PlayFabLoginAsync(email, password);
await pf.PlayFabGetLeaderboardAsync();
System.Threading.Thread.Sleep(2000);
await pf.PlayFabAddToLeaderboardAsync(gameScore);
System.Threading.Thread.Sleep(2000);
await pf.PlayFabGetLeaderboardAsync();
6.) Output:
Successful account creation.
Successful login! Your session ID is AF4A9784BAA8D47A--3355AEF3FDC01221-4EEEA-8D9650D3BDE6E3B-qtNQP7Itb7JZ1xDrDofAg2KVXBC9C2f/eez3z1VeTGo=
Successfully retrieved 2 leaderboard entries:
Player 9E944E78AA2F06A5 with 1000 points.
Player 7AF257A88CA152AD with 300 points.
Successfully added your score 1100 to the highscores leaderboard!
Successfully retrieved 3 leaderboard entries:
Player AF4A9204BAD7D47A with 1100 points.
Player 9E944E78AA2F06A5 with 1000 points.
Player 7AF257A88CA152AD with 300 points.
Press any key to continue . . .