Being a beginner in game development, I am finding this course really enjoyable. In our project of making a scratch game on our own, we learnt how to make a simple game on scratch. And here I will be sharing a tutorial based on how I made the game so that it can be helpful for other beginners in game development like me. The end product will look kinda like this.
Step by Step Procedure:
1) First we have to create our character who will jump over obstacles. To create our character, we can choose any sprite from scratch's library of sprites. I chose the cat.
2) Lets add a background for the cat to run in. For that, choose a backdrop. I chose the broadwalk.
3) Now lets move on to the coding part for the cat. We need the cat to jump. So choose the change y by 10 code from the Motions group. We need the cat to jump higher so we can take the repeat code from the Control group. Repeat these steps for a second time but edit the change y by 10 to change y by -10. We can then add when space key pressed from the Events group to make the cat jump when space-bar is pressed. We can make the cat more realistic by adding a meow sound effect from the Sound group.
4) Now lets create the obstacle, our cat needs to jump over. For that add another sprite from scratch's sprite library. I chose the apple as the cat's obstacle.
However we need the apple to be smaller in size than the cat so that it can jump over it. For that change the apple's size from 100 to 60.
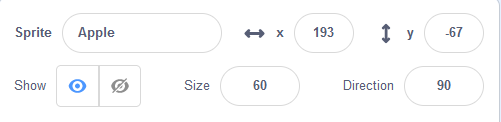
Now that looks better.
Keep the apple at the end of the screen where it will start its glide. Then take the go to x code from the Motion group. It will take the apple's coordinates as the go to point. Now place the apple at the other point of the screen where it will end its glide and import the glide 1 secs to x code from the Motion group. Now we need this to go on as long as the game isn't over so take the add the forever code from the Control group. Add the event when flag clicked from the Events group so that it starts as soon as the game starts.
5) Now we need the obstacles to actually act like obstacles. So add the codes stop all and wait until from the Control group. Then attach the code touching cat from the Sensing group. Add the when flag clicked code here as well so that it starts right from the beginning.
6) Go back to the cat sprite. We need to make the cat go back to its original place when the game ends. So put the cat at its starting position and add the code go to x from the Motion group. Add the code when flag clicked so that it starts right from the beginning.
7) Now duplicate the apple sprite for more obstacles.
Add the code wait 1 seconds from the Control group and hide it for some time and then show after the first obstacle is passed using the hide and show codes from the Looks group.
8) Now moving on to the most important and rewarding part of any game. Every game needs a scoring system. For that go the Variables group, make a variable and name it Score.
Take the code change score by 1 and attach it to the code of the cat sprite which controls the jumping. On other hand, take the code set score to 0 and add it to the code of the cat sprite which makes the game start over.
And thus our simple jumping game is ready!
I hope that this tutorial was useful to you. Thank you for reading the entire way.
References:
- Biswadeep Bhattacharjee (20BCG10025)
VIT Bhopal (CSE with specialization in Gaming Technology)