So creative! Good job :)
algiorgi
Creator of
Recent community posts
DAY 9
I want to make a mention about all the resources that I have used in this game. I think that the best place to do it is in the credits scene. So, I made a little change to the Level information to set a flag for the last level.
[CreateAssetMenu(fileName = "Level", menuName = "FreeThemAll/New Level", order = 1)] public class LevelData : ScriptableObject { public int Number; public string SceneNameFile; public int PrisonersCount; public int EnemiesCount; public bool Hashelp; public string HelpText; public bool IsTheLastLevel; }
It´s not the best way to do it, I know, but it works. Of course I had to change the Game Manager.
bool isTheLastLevel = _currentLevel != null && _currentLevel.IsTheLastLevel; _currentLevel = null; if (isTheLastLevel) { _state = GameState.SHOWING_CREDITS; SceneManager.LoadSceneAsync("Credits"); }
I have to reference the assets that I used
DAY 8
I had finished the first playable prototype so I uploaded a draft version of the game but I found that it never finished loading. So I did some research and I discovered that this is actually a problem with Unity WebGL. Here the post.
So if you are using Unity 2020 and you are developing for WebGL you have to disable compression in the Publishing Settings
DAY 7 CONTINUED
I think I have to add some explanation on how to play the game. Maybe it doesnt looks nice but it was quick and easy thing to do.
This kind of typing effect can be achieved with a Coroutine:
private IEnumerator DisplayHelpText(string text) { for (int i = 0; i < text.Length; i++) { char letter = text[i]; _textHelp.text += letter; yield return new WaitForSeconds(_displayCharacterSpeed); } _closeHelpTextButton.gameObject.SetActive(true); }
DAY 6
I changed a little the GameManager to add the ability of restart the game whe you die. And i had to add some functions to initialize things.
So, when the level is loaded the game starts
private void Play() { _currentLevel.StartLevel(); Instantiate(_playerPrefab, _currentLevel.PlayerStartingPoint.position, Quaternion.identity); _state = GameState.PLAYING_LEVEL; }
I guess I can let the level instantiate the player but for now I will do it in the GameManager. And when the player die, I just have to call Play again, without reloading the level.
PlayerController
private void OnTriggerEnter2D(Collider2D collision) { if (collision.gameObject.CompareTag("Enemy")) { GameManager.Instance.GameOver(); Destroy(gameObject); } }
GameManager
public void GameOver() { _state = GameState.RESTARTING_LEVEL; }
private void Update() { if (_state.Equals(GameState.PLAYING_LEVEL)) { if (_currentLevel.PrisonersCount == 0 && _currentLevel.IsTeleporterActive() == false) { _currentLevel.ActivateTeleporter(); } } else if (_state.Equals(GameState.RESTARTING_LEVEL)) { Play(); } }
I hope its work.
When all the prisoners are freed, the teleporter should be activatedWhen player die the level should be restarted
Next steps: UI
DAY 5
I said the next step was to destroy the enemies. But, I decide to freeze the enemies for a while instead of destroying them.
I'm not sure if its a good idea but it increases a little bit the game difficulty. You cannot destroy enemies and if they are frozen and you touch them, you lose.
The code to freeze enemies is quite simple, maybe there is a better way to do it:
public void Freeze() { if (_isFrozen) { return; } _isFrozen = true; _animator.SetFloat(_fSpeedHash, 0); StartCoroutine(Defrost()); } private IEnumerator Defrost() { yield return new WaitForSeconds(_defrostTime); _isFrozen = false; }
The player can fire and freeze enemies
DAY 3 CONTINUED
This is what the level component looks like:
I´m using Scriptable Objects for the different levels
I think it is enough to handle different levels. When I start to creating them, I will know if I have to change something
A level designer should be able to set how many prisoner a level hasA level designer should be able to set how many enemies a level hasA level designer should be able to set where the player should spawn
ENEMIES
I will try to do something simple for enemies. At least for the very first version of the game. The general idea is to set up a list of waypoints wich a enemy has to follow.
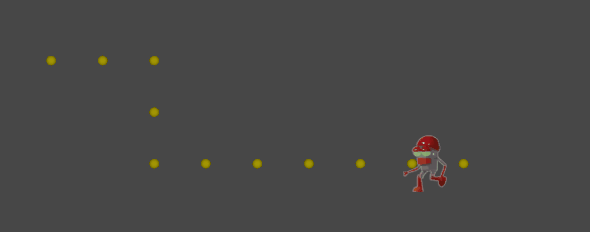
The yellow dots are each waypoint. You can draw something in a Unity Scene calling the OnDrawGizmos function. It is useful to have visual indicators of some objects in your game.
private void OnDrawGizmos() { for (int i = 0; i < _waypoints.Length; i++) { Gizmos.color = Color.yellow; Gizmos.DrawSphere(_waypoints[i], 0.1f); } }
Enemies can only patrol a specific area
I will start with the shooting behaviour the next day.
DAY 3
I should be able to load any level from 1 to x. When a level is loaded, I should instantiate the Player. This will be my initial aproach.
The GameManager will handle this kind of things
private void LoadNextLevel() { _currentLevel = null; _state = GameState.LOADING_LEVEL; AsyncOperation ao = SceneManager.LoadSceneAsync($"Level{_nextLevelToLoad}"); ao.completed += OnLevelLoaded; }
private void OnLevelLoaded(AsyncOperation ao) { ao.completed -= OnLevelLoaded; // Is it necessary? Level loadedLevel = FindObjectOfType<Level>(); if (loadedLevel == null) { throw new Exception("Could not find a level"); } _currentLevel = loadedLevel; Instantiate(_playerPrefab, loadedLevel.PlayerStartingPoint.position, Quaternion.identity); _state = GameState.PLAYING_LEVEL; }
The Level class holds some data like where the player should starts and how many prisoners the player has to free.
Its late here in Argentina and I have to work tomorrow. I will try to continue later :)
DAY 1 AND 2 CONTINUED
So, the player must free prisoners... but I dont have idea how to make the prisoners. I couldnt find any assets that I liked.
So this is the first version of the prisoners. And maybe the last.
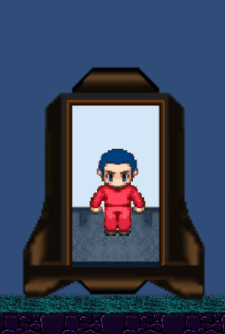
The weird movement is generated by a Sin function
private void AnimatePrisoner() { Vector3 temporalPosition = _prisoner.localPosition; temporalPosition.y = amplitude * Mathf.Sin(Time.time * frequency) + _verticalShift; _prisoner.localPosition = temporalPosition; }
The prisoner has a collider and when the player collides with it, the remaining prisoner count decreases.
private void OnTriggerEnter2D(Collider2D collision) { if (collision.gameObject.CompareTag("Player")) { FreePrisoner(); } }
Now I should start to work on the level desing, at least the logical desing
Introduction
First of all, sorry for my bad English. It Is not my native language so I´m sure I will make a lot of mistakes. Also, I don´t have much time to work on the Jam (I have a full time job as a Software Developer) but since it is my very first jam, I thought that would be nice try to write something about the process.
The Game
I´m not an artist. I don´t know how to use any graphic editor. So, I have to use as many free assest as I can.
The theme for this jam is Free. I thought to make a game about a kind of astronaut who uses a jetpack and tries to free some prisoners. When all the prisoners are freed, the player have to reach a teleporter to be teleported to the next level
I will use this free asset from the Unity Store.
Great, I have something. It may be silly or simple but I know I will learn a lot. I mean, that is my goal, learn.
So, I don't have much time and I don't have much experience making games... Mmm I should try to make a plan.
The MVP (Minimum Viable Product)
At least, I think that my game should have this features:
- The player can move from left to right using A and D
- The player can fly using W
- A walking animation should be played when the player is walking
- The jetpack would be animated when the player is flying
- The jetpack is unlimited
- A level designer should be able to set how many prisoner a level has
- A level designer should be able to set how many enemies a level has
- A level designer should be able to set where the player should spawn
- When all the prisoners are freed, the teleporter should be activated
- The UI should show the remaining prisoner count
- When the teleporter is active and the player reaches it, the Game Manager must load the next level
- Enemies can only patrol a specific area
- The player can fire and destroy enemies
- The player have infinite ammo
- The player can pause the game
- I have to reference the assets that I used
Wow... it is a lot. I hope to make it
DAY 1 AND 2
I worked on the player´s movement. It´s not perfect but I´m happy.
I think I can mark some features as done!